扫码阅读
手机扫码阅读
Odoo 集成 ElasticSearch

我们非常重视原创文章,为尊重知识产权并避免潜在的版权问题,我们在此提供文章的摘要供您初步了解。如果您想要查阅更为详尽的内容,访问作者的公众号页面获取完整文章。

神州数码云基地
扫码关注公众号
文章摘要
本文由数据开发工程师王泽宇撰写,主要内容是关于如何将Odoo的数据与ElasticSearch进行同步。
Step 1: 安装分词器
文章首先指导读者在ElasticSearch的bin目录下安装中文ik分词器,并提醒集群安装的情况下需要在每个节点安装。安装后需重启ElasticSearch服务。
Step 2: 测试分词器
接着,文章介绍了如何创建测试索引并验证分词器安装成功。通过输出分词结果来检查分词器是否工作正常。
同步Odoo数据与ElasticSearch
文章继续介绍了使用Python操作ElasticSearch的包elasticsearch7和字段映射的重要性。字段映射可以解决不同模型中同名字段导致的冲突。
实现ES工具类
作者详细说明了如何实现ElasticSearch工具类,包括创建索引、删除索引、处理字段映射及索引文档的方法。
同步数据方法
文章解释了如何同步PostgreSQL字段和ElasticSearch索引,包括插入更新字段和删除字段的操作。
重写Odoo模型方法
为了同步增删改操作,需要重写Odoo模型的创建、修改和删除方法,并注意直接操作数据库会跳过同步过程。
重建索引与全文搜索实现
最后,文章提出了一种强制重建索引的方法,并实现了全文搜索的功能,包括对搜索结果的处理和封装。
整体而言,文章详细介绍了如何在ElasticSearch中进行中文分词、如何同步Odoo数据、以及如何进行全文搜索,以确保ElasticSearch索引与PostgreSQL数据库保持同步和一致性。
想要了解更多内容?

神州数码云基地
扫码关注公众号
神州数码云基地的其他文章
加入社区微信群
与行业大咖零距离交流学习
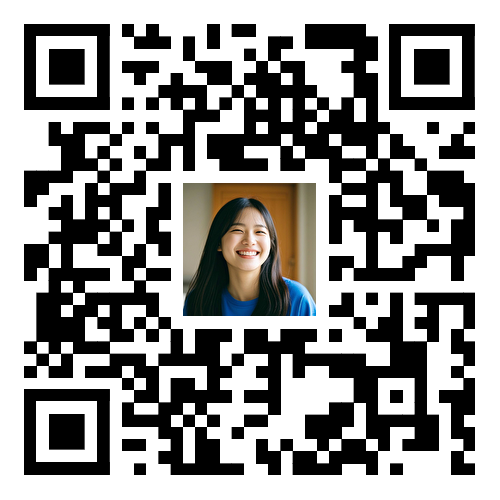
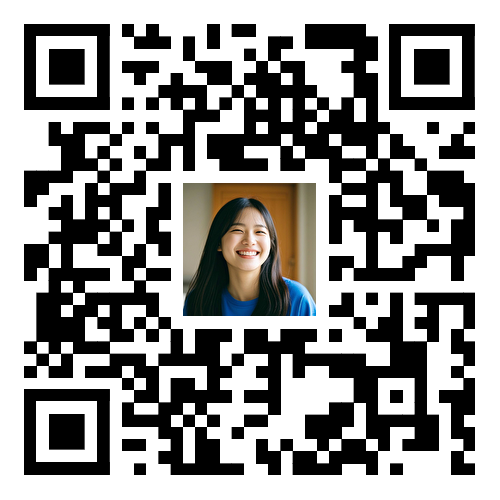
PMO实践白皮书
白皮书上线
白皮书上线