Python编写函数的基本原则和技巧

我们非常重视原创文章,为尊重知识产权并避免潜在的版权问题,我们在此提供文章的摘要供您初步了解。如果您想要查阅更为详尽的内容,访问作者的公众号页面获取完整文章。


Function Best Practices in Python
Functions are central to Python programming for organizing code and encapsulating logic. Efficient and well-structured functions enhance readability, reusability, and maintainability of code.
Python Function Principles
- Single Responsibility Principle: A function should handle a single task, making it easier to understand and reuse.
- Modular Design: Functions should act as modular components of the program, each solving one problem completely, reducing coupling and increasing maintainability.
- No Side Effects: Functions ideally should not alter input states or produce side effects, making them predictable and testable.
Functions' Purposes
- Code Reuse and Decoupling: Functions encapsulate shared features for reuse in different parts, reducing code duplication and interactions between code blocks for better modularity.
- Divide and Conquer: Functions allow developers to break complex problems into manageable chunks, simplifying solutions and clarifying code organization.
- Abstraction and Interface Consistency: Functions abstract complexity, allowing developers to use features without knowledge of internal details, while consistent interfaces make function usage more intuitive.
Tips for Writing Functions
- Clear Naming: Use descriptive names that accurately reflect the function's purpose, which is key for self-documenting code.
- Type Annotations: Python's type annotation feature can specify types for parameters and return values, aiding understanding and allowing for static type checking.
- Reasonable Parameter Quantity: Keep the number of parameters minimal to avoid errors and unclear function responsibilities.
- Documentation Strings: Provide detailed docstrings for function purposes, parameters, return values, and potential side effects, crucial for maintenance and collaboration.
- Avoid Global Variables: Minimize the use of global variables to prevent unpredictable behaviors and increased coupling.
- Error Handling: Manage errors effectively using exception handling mechanisms to avoid program crashes.
- Utilize Python Features: Leverage Python features like list comprehensions, generators, and decorators for concise and efficient coding.
Examples of Common Algorithmic Functions
The article provides examples of common algorithmic functions like the binary search and Fibonacci sequence calculation, illustrating the implementation of best practices in function writing.
Binary Search Algorithm
The binary search algorithm is implemented recursively, taking a sorted list, a target value, and search boundaries as parameters and returning the index of the target or -1 if not found.
Fibonacci Sequence Calculation
The Fibonacci sequence calculation is described as a recursive function that takes an integer n and returns the nth value of the sequence.
想要了解更多内容?


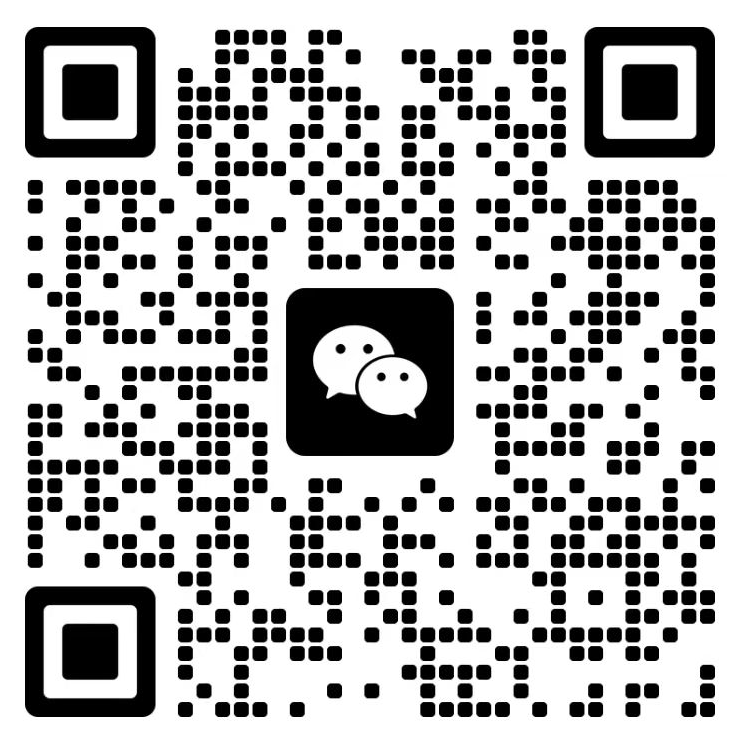
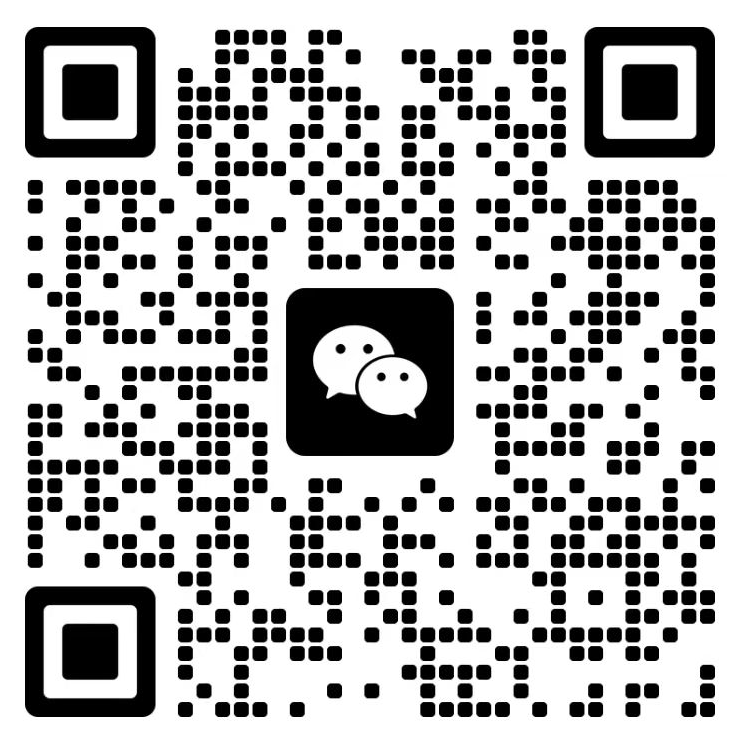